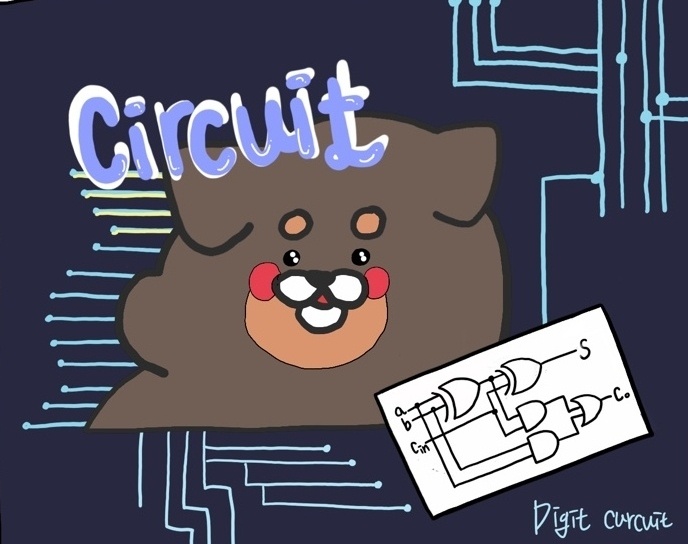
Block Diamgram
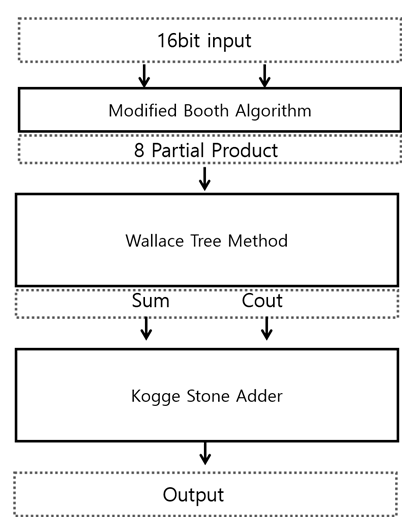
Structure
Modified Booth Algorithm
X_sel, X_2SEL, neg 신호 생성
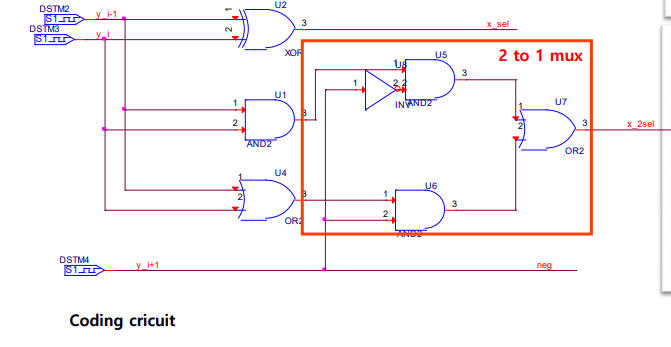
Partial Product 생성
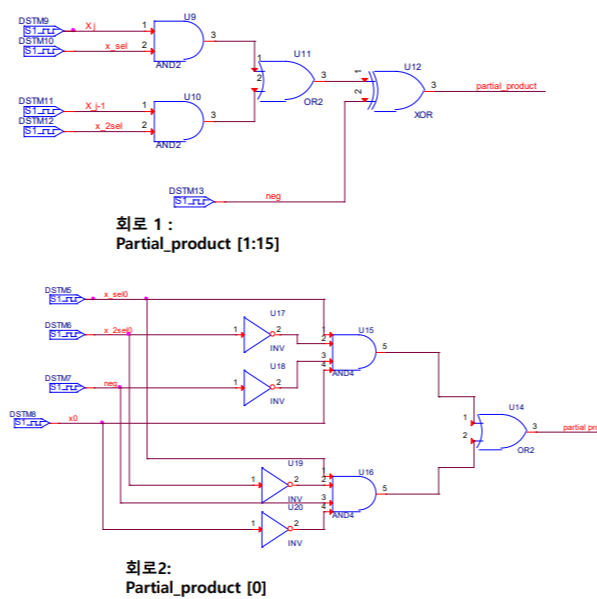
make the 1(x_sel=1 , neg=1), 2(x_2sel=1, neg=1) to 2'complement module
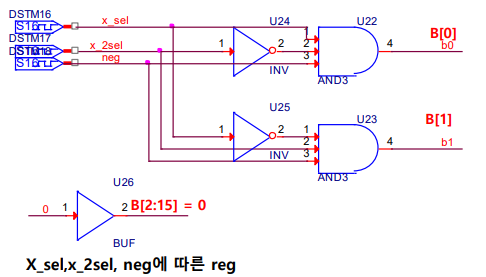
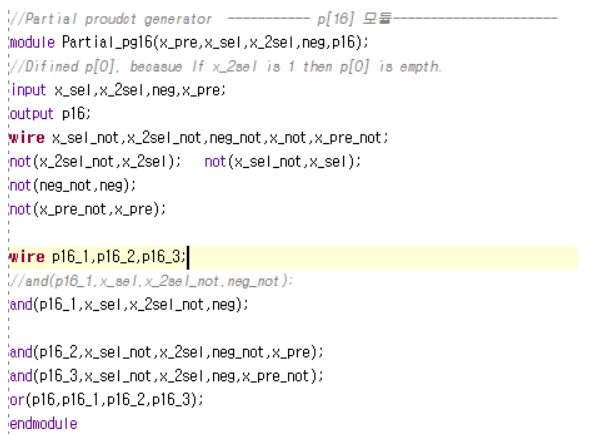
Wallace Tree-CSA
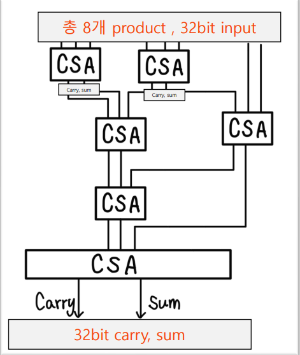
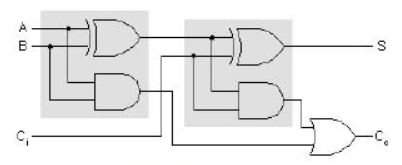
ci자리에 입력 C를 넣고 C0는 propagation이 아닌 output으로 나가게 하기.
이를 32bit 병렬연결.
Kogge Stone adder
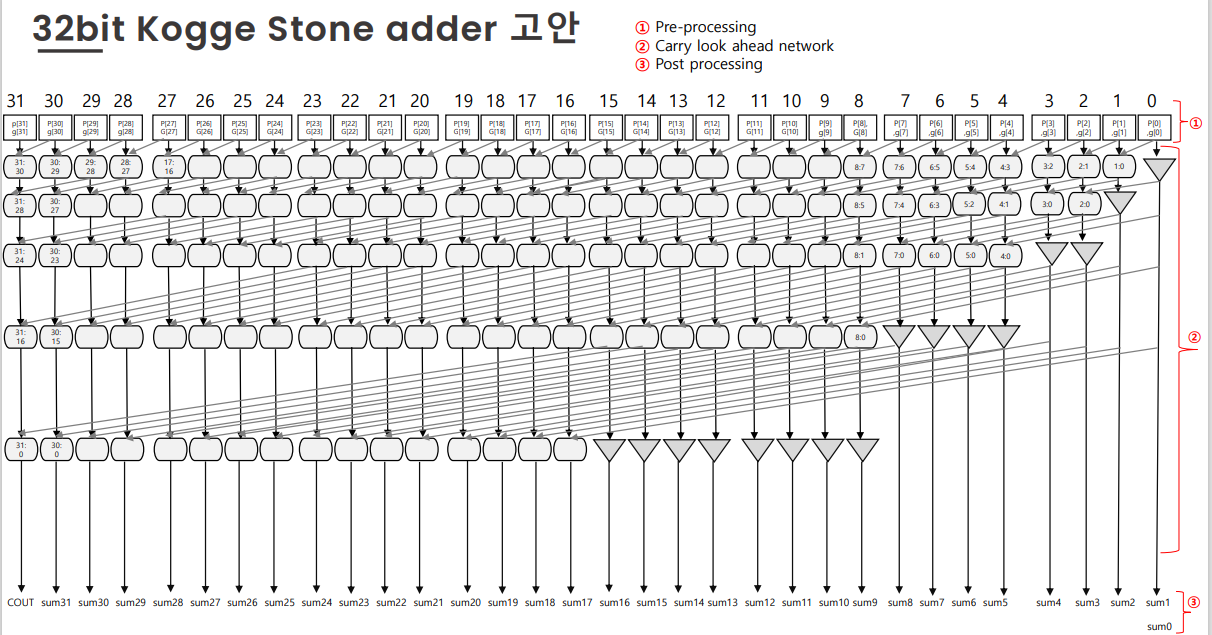
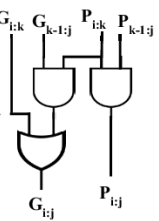
Code
module buterfly_multiplier(x,y,sum);
input [15:0] x,y;
output [31:0] sum;
wire cout;
wire [31:0] pp1,pp2,pp3,pp4,pp5,pp6,pp7,pp8;
Modified_booth_algorithm (x,y,pp1,pp2,pp3,pp4,pp5,pp6,pp7,pp8);
wire [31:0] Wallace_sum,Wallace_cout;
Wallace_tree Wallace(pp1,pp2,pp3,pp4,pp5,pp6,pp7,pp8,Wallace_sum,Wallace_cout);
Kogge_stone_adder Kogge_Stone(Wallace_sum,Wallace_cout,sum,cout);
endmodule
module Modified_booth_algorithm (x,y,pp1,pp2,pp3,pp4,pp5,pp6,pp7,pp8);
input [15:0] x,y;
output [31:0] pp1,pp2,pp3,pp4,pp5,pp6,pp7,pp8;
wire [16:0] product1,product2,product3,product4,product5,product6,product7,product8;
wire [7:0] x_sel,x_2sel,neg;
wire [16:0] product_1,product_2,product_3,product_4,product_5,product_6,product_7,product_8;
booth_coding booth1(x,y,product1,product2,product3,product4,product5,product6,product7,product8,x_sel,x_2sel,neg);
two_com twocom(product1,product2,product3,product4,product5,product6,product7,product8,x_sel,x_2sel,neg,product_1,product_2,product_3,product_4,product_5,product_6,product_7,product_8);
booth_algorithm booth(product_1,product_2,product_3,product_4,product_5,product_6,product_7,product_8, pp1,pp2,pp3,pp4,pp5,pp6,pp7,pp8);
endmodule
//----------------------product 32bit 만들기 ----------------------
module booth_algorithm(p1,p2,p3,p4,p5,p6,p7,p8,product1,product2,product3,product4,product5,product6,product7,product8);
input [16:0] p1,p2,p3,p4,p5,p6,p7,p8;
output [31:0] product1,product2,product3,product4,product5,product6,product7,product8;
assign product1 [31:0] = {{15 {p1[16] }},p1[16:0]};
assign product2 [31:0] = {{13 { p2[16] }},p2[16:0],2'b0};
assign product3 [31:0] = {{11 { p3[16] }},p3[16:0],4'b0};
assign product4 [31:0] = {{9 { p4[16] }},p4[16:0],6'b0};
assign product5 [31:0] = {{7 { p5[16] }},p5[16:0],8'b0};
assign product6 [31:0] = {{5 { p6[16] }},p6[16:0],10'b0};
assign product7 [31:0] = {{3 { p7[16] }},p7[16:0],12'b0};
assign product8 [31:0] = {p8[16],p8[16:0],14'b0};
endmodule
//----------------------------Part circuit coding -----------------------------------------------------------------------------------------
//Coding_circuit ..............................x_sel,x_2sel 신호 만들기
module coding_circuit(pre_y,y,next_y,x_sel,x_2sel,neg);
input pre_y,y,next_y;
output x_sel,x_2sel,neg;
//coding circuit
//x_sel,x_2sel genearate
xor(x_sel,pre_y,y);
wire L,H,H_temp;
and(L,pre_y,y); or(H_temp,pre_y,y); not(H,H_temp);
mux2_to_1 mux(L,H,x_2sel,next_y);
buf(neg,next_y);
endmodule
//-----------------------mux2_to_1-------------------------------------
module mux2_to_1(i0,i1,out,s);
input i0,i1,s;
output out;
wire s_not;
not(s_not,s);
wire w1,w2;
and(w1,i0,s_not);
and(w2,i1,s);
or(out,w1,w2);
endmodule
//Partial proudct generator ----------- p[0] 모듈----------------------
module Partial_pg0(x,x_sel,x_2sel,neg,p0);
//Difined p[0], becasue If x_2sel is 1 then p[0] is empth.
input x,x_sel,x_2sel,neg;
output p0;
wire x_sel_not,x_2sel_not,neg_not,x_not;
not(x_not,x);
not(x_2sel_not,x_2sel);
not(neg_not,neg);
wire p0_1,p0_2,p0_3;
and(p0_1,x_sel,x_2sel_not,neg_not,x);
and(p0_2,x_sel,x_2sel_not,neg,x_not);
and(p0_3,x_sel_not,x_2sel_not,neg);
or(p0,p0_1,p0_2);
endmodule
//Partial proudct generator-------------x [ 1: 15 ] , p [1: 15] 생성----------------
module Partial_pg(x,pre_x,x_sel,x_2sel,neg,pp);
//Defined p[ 1 : 15 ] using x [ 1: 15 ]
input x,pre_x;
input x_sel,x_2sel,neg;
output pp;
wire xj,xj_1,or_x;
and(xj,x,x_sel); and(xj_1,pre_x,x_2sel); or(or_x,xj,xj_1);
wire pp_temp1;
xor(pp_temp1,or_x,neg);
wire x_sel_not,x_2sel_not,pp_temp2,pp_temp3;
not(x_sel_not,x_sel); not(x_2sel_not,x_2sel); and(pp_temp2,x_sel_not,x_2sel_not,neg);
not(pp_temp3,pp_temp2); and(pp,pp_temp3,pp_temp1);
endmodule
//Partial proudct generator ----------- p[16] 모듈----------------------
module Partial_pg16(x_pre,x_sel,x_2sel,neg,p16);
//Difined p[0], becasue If x_2sel is 1 then p[0] is empth.
input x_sel,x_2sel,neg,x_pre;
output p16;
wire x_sel_not,x_2sel_not,neg_not,x_not,x_pre_not;
not(x_2sel_not,x_2sel); not(x_sel_not,x_sel);
not(neg_not,neg);
not(x_pre_not,x_pre);
wire p16_1,p16_11,p16_2,p16_3;
and(p16_11,x_sel,x_2sel_not,neg_not,x_pre);
and(p16_1,x_sel,x_2sel_not,neg,x_pre_not);
and(p16_2,x_sel_not,x_2sel,neg_not,x_pre);
and(p16_3,x_sel_not,x_2sel,neg,x_pre_not);
or(p16,p16_11,p16_1,p16_2,p16_3);
endmodule
// 16bit pg ---------------------pg 76bit으로 합치기----------------------------------------//////////////////////
module pg_16bit(x,x_sel,x_2sel,neg,pp);
input x_sel,x_2sel,neg;
input [15:0] x;
output [16:0] pp;
//Generate p [ 0 : 15]
Partial_pg0 pg0(x[0],x_sel,x_2sel,neg,pp[0]);
Partial_pg pg1(x[1],x[0],x_sel,x_2sel,neg,pp[1]); Partial_pg pg2(x[2],x[1],x_sel,x_2sel,neg,pp[2]);
Partial_pg pg3(x[3],x[2],x_sel,x_2sel,neg,pp[3]); Partial_pg pg4(x[4],x[3],x_sel,x_2sel,neg,pp[4]);
Partial_pg pg5(x[5],x[4],x_sel,x_2sel,neg,pp[5]); Partial_pg pg6(x[6],x[5],x_sel,x_2sel,neg,pp[6]);
Partial_pg pg7(x[7],x[6],x_sel,x_2sel,neg,pp[7]); Partial_pg pg8(x[8],x[7],x_sel,x_2sel,neg,pp[8]);
Partial_pg pg9(x[9],x[8],x_sel,x_2sel,neg,pp[9]); Partial_pg pg10(x[10],x[9],x_sel,x_2sel,neg,pp[10]);
Partial_pg pg11(x[11],x[10],x_sel,x_2sel,neg,pp[11]); Partial_pg pg12(x[12],x[11],x_sel,x_2sel,neg,pp[12]);
Partial_pg pg13(x[13],x[12],x_sel,x_2sel,neg,pp[13]); Partial_pg pg14(x[14],x[13],x_sel,x_2sel,neg,pp[14]);
Partial_pg pg15(x[15],x[14],x_sel,x_2sel,neg,pp[15]); Partial_pg16 pg16(x[15],x_sel,x_2sel,neg,pp[16]);
endmodule
//coding, parital product circuit module 합치기
module booth_coding(x,y,product1,product2,product3,product4,product5,product6,product7,product8,x_sel,x_2sel,neg);
input [15:0] x,y;
output[16:0] product1,product2,product3,product4,product5,product6,product7,product8;
output [7:0] x_sel,x_2sel,neg;
// 3bit pair and generate x_sel,x_2sel,neg by coding_circuit
coding_circuit c1(0,y[0],y[1],x_sel[0],x_2sel[0],neg[0]); coding_circuit c2(y[1],y[2],y[3],x_sel[1],x_2sel[1],neg[1]);
coding_circuit c3(y[3],y[4],y[5],x_sel[2],x_2sel[2],neg[2]); coding_circuit c4(y[5],y[6],y[7],x_sel[3],x_2sel[3],neg[3]);
coding_circuit c5(y[7],y[8],y[9],x_sel[4],x_2sel[4],neg[4]); coding_circuit c6(y[9],y[10],y[11],x_sel[5],x_2sel[5],neg[5]);
coding_circuit c7(y[11],y[12],y[13],x_sel[6],x_2sel[6],neg[6]); coding_circuit c8(y[13],y[14],y[15],x_sel[7],x_2sel[7],neg[7]);
//Generate partial product generator, product
pg_16bit pg_1(x,x_sel[0],x_2sel[0],neg[0],product1); pg_16bit pg_2(x,x_sel[1],x_2sel[1],neg[1],product2);
pg_16bit pg_3(x,x_sel[2],x_2sel[2],neg[2],product3); pg_16bit pg_4(x,x_sel[3],x_2sel[3],neg[3],product4);
pg_16bit pg_5(x,x_sel[4],x_2sel[4],neg[4],product5); pg_16bit pg_6(x,x_sel[5],x_2sel[5],neg[5],product6);
pg_16bit pg_7(x,x_sel[6],x_2sel[6],neg[6],product7); pg_16bit pg_8(x,x_sel[7],x_2sel[7],neg[7],product8);
endmodule
//---------------------------------------------------------------------보수 circuit module ---------------------------------------------------------
//---------------2'complement. +1 하기. 하지만 한칸 밀린경우 +2하기.
module two_com(pp1,pp2,pp3,pp4,pp5,pp6,pp7,pp8,x_sel,x_2sel,neg,product_1,product_2,product_3,product_4,product_5,product_6,product_7,product_8);
input [7:0] x_sel,x_2sel,neg;
input [16:0]pp1,pp2,pp3,pp4,pp5,pp6,pp7,pp8;
output [16:0]product_1,product_2,product_3,product_4,product_5,product_6,product_7,product_8;
wire [16:0] b1,b2,b3,b4,b5,b6,b7,b8;
pluse two1(x_sel[0],x_2sel[0],neg[0],b1); pluse two2(x_sel[1],x_2sel[1],neg[1],b2);
pluse two3(x_sel[2],x_2sel[2],neg[2],b3); pluse two4(x_sel[3],x_2sel[3],neg[3],b4);
pluse two5(x_sel[4],x_2sel[4],neg[4],b5); pluse two6(x_sel[5],x_2sel[5],neg[5],b6);
pluse two7(x_sel[6],x_2sel[6],neg[6],b7); pluse two8(x_sel[7],x_2sel[7],neg[7],b8);
adder_17bit adder1(pp1,b1,product_1); adder_17bit adder2(pp2,b2,product_2);
adder_17bit adder3(pp3,b3,product_3); adder_17bit adder4(pp4,b4,product_4);
adder_17bit adder5(pp5,b5,product_5); adder_17bit adder6(pp6,b6,product_6);
adder_17bit adder7(pp7,b7,product_7); adder_17bit adder8(pp8,b8,product_8);
endmodule
//module 2'complement의 +1 or +2 신호 만들기.
module pluse(x_sel,x_2sel,neg,b);
input x_sel,x_2sel,neg;
output[16:0] b;
wire x_2sel_not,x_sel_not; not(x_2sel_not,x_2sel); not(x_sel_not,x_sel);
assign b[16:0] = {14'b0, (x_sel_not) &( x_2sel )&( neg) , x_sel&x_2sel_not&neg };
endmodule
//16bit adder----------------보수 시 덧셈------------------------------------------
module adder_17bit (a,b,sum);
input [16:0] a,b;
output [16:0] sum;
wire c_out;
wire [16:0] c;
FullAdder adder0(a[0],b[0],0,sum[0],c[0]); FullAdder adder1(a[1],b[1],c[0],sum[1],c[1]);
FullAdder adder2(a[2],b[2],c[1],sum[2],c[2]); FullAdder adder3(a[3],b[3],c[2],sum[3],c[3]);
FullAdder adder4(a[4],b[4],c[3],sum[4],c[4]); FullAdder adder5(a[5],b[5],c[4],sum[5],c[5]);
FullAdder adder6(a[6],b[6],c[5],sum[6],c[6]); FullAdder adder7(a[7],b[7],c[6],sum[7],c[7]);
FullAdder adder8(a[8],b[8],c[7],sum[8],c[8]); FullAdder adder9(a[9],b[9],c[8],sum[9],c[9]);
FullAdder adder10(a[10],b[10],c[9],sum[10],c[10]); FullAdder adder11(a[11],b[11],c[10],sum[11],c[11]);
FullAdder adder12(a[12],b[12],c[11],sum[12],c[12]); FullAdder adder13(a[13],b[13],c[12],sum[13],c[13]);
FullAdder adder14(a[14],b[14],c[13],sum[14],c[14]); FullAdder adder15(a[15],b[15],c[14],sum[15],c[15]);
FullAdder adder16(a[16],b[16],c[15],sum[16],c_out);
endmodule
//1bit fulladder--------------------------------------------------------------------------
module FullAdder(
input x, input y, input cin,
output sum,output cout
);
wire c1,c2,c3;
xor(sum,x,y,cin);
and(c1,x,y),(c2,x,cin),(c3,y,cin);
or(cout,c1,c2,c3);
endmodule
/*
module CSA_32bit(x,y,z,sum,cout);
input [31:0]x,y,z;
output [31:0]sum;
output [31:0]cout;
assign cout[0]=0;
Full_adder_16bit fa16_1(x[15:0],y[15:0],z[15:0],sum[15:0],cout[16:1]);
Full_adder_16bit fa16_2(x[31:16],y[31:16],z[31:16],sum[31:16],cout[31:17]);
endmodule
module Full_adder_16bit(x,y,z,sum,cout);
input [15:0] x,y,z;
output [15:0] sum;
output [15:0]cout;
Full_Adder_8bit fad1(x[7:0],y[7:0],z[7:0],sum[7:0],cout[7:0]);
Full_Adder_8bit fad2(x[15:8],y[15:8],z[15:8],sum[15:8],cout[15:8]);
endmodule
module Full_Adder_8bit(x,y,z,sum,cout);
input [7:0] x,y,z;
output [7:0] sum;
output [7:0] cout;
FullAdder f1(x[0],y[0],z[0],sum[0],cout[0]);
FullAdder f2(x[1],y[1],z[1],sum[1],cout[1]);
FullAdder f3(x[2],y[2],z[2],sum[2],cout[2]);
FullAdder f4(x[3],y[3],z[3],sum[3],cout[3]);
FullAdder f5(x[4],y[4],z[4],sum[4],cout[4]);
FullAdder f6(x[5],y[5],z[5],sum[5],cout[5]);
FullAdder f7(x[6],y[6],z[6],sum[6],cout[6]);
FullAdder f8(x[7],y[7],z[7],sum[7],cout[7]);
endmodule*/
//1bit CSA-------------------------------------------------------------------------------------------------
module CSA_1bit(a,b,c,sum,cout);
input a,b,c;
output sum,cout;
wire w1,w2,w3,w4,w5;
wire a_not,b_not,c_not; not(a_not,a); not(b_not,b); not(c_not,c);
wire w11,w22,w33;
wire ab,ac,bc;
xor(w1,b,c);
and(w2,w1,a_not); and(w3,a,b,c); and(w4,a,b_not,c_not); or(sum,w2,w3,w4);
and(w11,b,c);and(w22,a,b); and(w33,a,c);
or(cout,w11,w22,w33);
endmodule
//8bit CSA_CIN-------------------------------------------------------------------------------------------------
module CSA_8bit_cin(product1,product2,product3,sum,cout);
input [6:0] product1,product2,product3;
output [7:0] sum, cout;
buf(cout[0],0); //cout[0]=0
//cout이 하나씩 밀려 carry역할을 함.
CSA_1bit CSA1(product1[0],product2[0],product3[0],sum[0],cout[1]); CSA_1bit CSA2(product1[1],product2[1],product3[1],sum[1],cout[2]);
CSA_1bit CSA3(product1[2],product2[2],product3[2],sum[2],cout[3]); CSA_1bit CSA4(product1[3],product2[3],product3[3],sum[3],cout[4]);
CSA_1bit CSA5(product1[4],product2[4],product3[4],sum[4],cout[5]); CSA_1bit CSA6(product1[5],product2[5],product3[5],sum[5],cout[6]);
CSA_1bit CSA7(product1[6],product2[6],product3[6],sum[6],cout[7]);
endmodule
//8bit CSA_Cout-------------------------------------------------------------------------------------------------
module CSA_8bit_cout(product1,product2,product3,sum,cout);
input [6:0] product1,product2,product3;
output [7:0] sum, cout;
//cout하나씩 밀기
CSA_1bit CSA1(product1[0],product2[0],product3[0],sum[0],cout[1]); CSA_1bit CSA2(product1[1],product2[1],product3[1],sum[1],cout[2]);
CSA_1bit CSA3(product1[2],product2[2],product3[2],sum[2],cout[3]); CSA_1bit CSA4(product1[3],product2[3],product3[3],sum[3],cout[4]);
CSA_1bit CSA5(product1[4],product2[4],product3[4],sum[4],cout[5]); CSA_1bit CSA6(product1[5],product2[5],product3[5],sum[5],cout[6]);
CSA_1bit CSA7(product1[6],product2[6],product3[6],sum[6],cout[7]);
endmodule
//------------------------------------- 32bit CSA---------------------------------------------------------
module CSA_32bit(product1,product2,product3,sum,cout);
input [31:0] product1,product2,product3;
output [31:0] sum, cout;
CSA_8bit_cin csa1(product1[6:0],product2[6:0],product3[6:0],sum[7:0],cout[7:0]);
CSA_8bit_cout csa2(product1[13:7],product2[13:7],product3[13:7],sum[14:7],cout[14:7]);
CSA_8bit_cout csa3(product1[20:14],product2[20:14],product3[20:14],sum[21:14],cout[21:14]);
CSA_8bit_cout csa4(product1[27:21],product2[27:21],product3[27:21],sum[28:21],cout[28:21]);
CSA_1bit CSA5(product1[28],product2[28],product3[28],sum[28],cout[29]);
CSA_1bit CSA6(product1[29],product2[29],product3[29],sum[29],cout[30]);
CSA_1bit CSA7(product1[30],product2[30],product3[30],sum[30],cout[31]);
wire w1,w2,w3,w4;
wire product1_not,product2_not,product3_not; not(product1_not,product1[31]); not(product2_not,product2[31]); not(product3_not,product3[31]);
xor(w1,product2[31],product3[31]);
and(w2,w1,product1_not); and(w3,product1[31],product2[31],product3[31]); and(w4,product1[31],product2_not,product3_not);
or(sum[31],w2,w3,w4);
endmodule
//------------------------------------- Wallace_tree---------------------------------------------------------
module Wallace_tree(product1,product2,product3,product4,product5,product6,product7,product8,sum,cout);
input [31:0] product1,product2,product3,product4,product5,product6,product7,product8;
output [31:0]sum,cout;
wire [31:0] cout1,cout2,cout3,cout4,cout5;
wire [31:0 ] sum1,sum2,sum3,sum4,sum5;
CSA_32bit cas_32bit1(product1,product2,product3,sum1,cout1);
CSA_32bit cas_32bit2(product4,product5,product6,sum2,cout2);
CSA_32bit cas_32bit3(product7,product8,sum2,sum4,cout4);
CSA_32bit cas_32bit4(cout1,sum1,cout2,sum3,cout3);
CSA_32bit cas_32bit5(cout3,sum3,cout4,sum5,cout5);
CSA_32bit cas_32bit6(cout5,sum5,sum4,sum,cout);
endmodule
//-----------------------------------Kogge-Stone-Adder ---------------------------------------
module Kogge_stone_adder(Wallace_sum,Wallace_cout,Kogge_sum,Kogge_cout );
input [31:0] Wallace_sum,Wallace_cout;
output [31:0] Kogge_sum;
output Kogge_cout;
wire [31:0] p,g,final_g;
Pre_processing pe_process(Wallace_sum,Wallace_cout,p,g);
CLA_network cla_net(p,g,final_g);
Post_processing post_process(p,final_g,Kogge_sum,Kogge_cout);
endmodule
//----------------------------------Pre_Processing ---------------------------------------
module Pre_processing(sum,cout,p,g);
input [31:0] sum, cout;
output [31:0] p,g;
PG_make pgg1(sum[7:0],cout[7:0],p[7:0],g[7:0]);
PG_make pgg2(sum[15:8],cout[15:8],p[15:8],g[15:8]);
PG_make pgg3(sum[23:16],cout[23:16],p[23:16],g[23:16]);
PG_make pgg4(sum[31:24],cout[31:24],p[31:24],g[31:24]);
endmodule
//pg 생성 8bit
module PG_make(sum,cout,p,g);
input [7:0] sum,cout; output [7:0] p,g;
xor(p[0],sum[0],cout[0]);xor(p[1],sum[1],cout[1]);xor(p[2],sum[2],cout[2]);xor(p[3],sum[3],cout[3]);
xor(p[4],sum[4],cout[4]);xor(p[5],sum[5],cout[5]);xor(p[6],sum[6],cout[6]);xor(p[7],sum[7],cout[7]);
and(g[0],sum[0],cout[0]);and(g[1],sum[1],cout[1]);and(g[2],sum[2],cout[2]);and(g[3],sum[3],cout[3]);
and(g[4],sum[4],cout[4]);and(g[5],sum[5],cout[5]);and(g[6],sum[6],cout[6]);and(g[7],sum[7],cout[7]);
endmodule
//----------------------------------CLA-Networt ---------------------------------------
module CLA_network(p,g,final_g);
input [31:0] p,g;
output [31:0] final_g;
wire [31:0] p1,g1,p2, g2,p3,g3 ,p4,g4,p5,g5;
wire gw1,gw2,gw3;
//-----------------첫번째 단 pg 연결, 생성------------------------------------------
buf(p1[0],p[0]); buf(g1[0],g[0]);
kogge1_8bit pair1_1(p[7:0],g[7:0],p1[7:1],g1[7:1]); kogge1_8bit pair1_2(p[14:7],g[14:7],p1[14:8],g1[14:8]);
kogge1_8bit pair1_3(p[21:14],g[21:14],p1[21:15],g1[21:15]); kogge1_8bit pair1_4(p[28:21],g[28:21],p1[28:22],g1[28:22]);
and(p1[29],p[29],p[28]); and(p1[30],p[30],p[29]); and(p1[31],p[31],p[30]);
and(gw1,p[29],g[28]); and(gw2,p[30],g[29]);and(gw3,p[31],g[30]);
or(g1[29],g[29],gw1); or(g1[30],g[30],gw2); or(g1[31],g[31],gw3);
//-----------------두번째 단 pg 연결, 생성------------------------------------------
wire gw4,gw5,gw6,gw7;
buf(p2[1],p1[1]); buf(g2[1],g1[1]);
kogge2_8bit pair2_1(p1[7:0],g1[7:0],p2[7:2],g2[7:2]); kogge2_8bit pair2_2(p1[13:6],g1[13:6],p2[13:8],g2[13:8]);
kogge2_8bit pair2_3(p1[19:12],g1[19:12],p2[19:14],g2[19:14]); kogge2_8bit pair2_4(p1[25:18],g1[25:18],p2[25:20],g2[25:20]);
kogge2_8bit pair2_5(p1[31:24],g1[31:24],p2[31:26],g2[31:26]);
//-----------------세번째 단 pg 연결, 생성------------------------------------------
buf(p3[2],p2[2]); buf(g3[2],g2[2]);
buf(p3[3],p2[3]); buf(g3[3],g2[3]);
kogge3_8bit pair3_1({p2[7:1],p1[0]},{g2[7:1],g1[0]}, p3[7:4],g3[7:4]); kogge3_8bit pair3_2(p2[11:4],g2[11:4],p3[11:8],g3[11:8]);
kogge3_8bit pair3_3(p2[15:8],g2[15:8],p3[15:12],g3[15:12]); kogge3_8bit pair3_4(p2[19:12],g2[19:12],p3[19:16],g3[19:16]);
kogge3_8bit pair3_5(p2[23:16],g2[23:16],p3[23:20],g3[23:20]); kogge3_8bit pair3_6(p2[27:20],g2[27:20],p3[27:24],g3[27:24]);
kogge3_8bit pair3_7(p2[31:24],g2[31:24],p3[31:28],g3[31:28]);
//-----------------네번째 단 pg 연결, 생성------------------------------------------
buf(p4[4],p3[4]); buf(g4[4],g3[4]); buf(p4[5],p3[5]); buf(g4[5],g3[5]);
buf(p4[6],p3[6]); buf(g4[6],g3[6]); buf(p4[7],p3[7]); buf(g4[7],g3[7]);
kogge4_16bit pair4_1({p3[15:4],p3[3:2],p2[1],p1[0]},{g3[15:4],g3[3:2],g2[1],g1[0]},p4[15:8],g4[15:8]); kogge4_16bit pair4_2(p3[23:8],g3[23:8],p4[23:16],g4[23:16]);
kogge4_16bit pair4_3(p3[31:16],g3[31:16],p4[31:24],g4[31:24]);
//-----------------다섯번째 단 pg 연결, 생성------------------------------------------
buf(p5[8],p4[8]); buf(g5[8],g4[8]); buf(p5[9],p4[9]); buf(g5[9],g4[9]);
buf(p5[10],p4[10]); buf(g5[10],g4[10]); buf(p5[11],p4[11]); buf(g5[11],g4[11]);
buf(p5[12],p4[12]); buf(g5[12],g4[12]); buf(p5[13],p4[13]); buf(g5[13],g4[13]);
buf(p5[14],p4[14]); buf(g5[14],g4[14]); buf(p5[15],p4[15]); buf(g5[15],g4[15]);
kogge5_32bit pair32({p4[31:8],p4[7:4],p3[3:2],p2[1],p1[0]},{g4[31:8],g4[7:4],g3[3:2],g2[1],g1[0]},p5[31:16],g5[31:16]);
assign final_g={g5[31:8],g4[7:4],g3[3:2],g2[1],g1[0]};
endmodule
//---------------3. Post processing. 32bit sum
module Post_processing(p,g5,sum,cout);
input [31:0] p,g5;
output [31:0] sum;
output cout;
buf(sum[0],p[0]);
//assign {sum,cout}= p^{g5[30:0],p[0]};
pgxor_8bit pg_pair1(p[7:0],g5[7:0],sum[7:1]);
pgxor_8bit pg_pair2(p[14:7],g5[14:7],sum[14:8]);
pgxor_8bit pg_pair3(p[21:14],g5[21:14],sum[21:15]);
pgxor_8bit pg_pair4(p[28:21],g5[28:21],sum[28:22]);
xor(sum[29],p[29],g5[28]);xor(sum[30],p[30],g5[29]);xor(sum[31],p[31],g5[30]);
buf(cout,g5[31]);
endmodule
//첫번째 단 연결 8bit---------------------------------------------
module kogge1_8bit(p,g,p1,g1);
input [7:0] p,g; output [7:1] p1,g1;
wire [7:0] gw;
and(p1[1],p[1],p[0]);
and(gw[0],p[1],g[0]); or(g1[1],gw[0],g[1]);
and(p1[2],p[2],p[1]);
and(gw[1],p[2],g[1]); or(g1[2],gw[1],g[2]);
and(p1[3],p[3],p[2]);
and(gw[2],p[3],g[2]); or(g1[3],gw[2],g[3]);
and(p1[4],p[4],p[3]);
and(gw[3],p[4],g[3]); or(g1[4],gw[3],g[4]);
and(p1[5],p[5],p[4]);
and(gw[4],p[5],g[4]); or(g1[5],gw[4],g[5]);
and(p1[6],p[6],p[5]);
and(gw[5],p[6],g[5]); or(g1[6],gw[5],g[6]);
and(p1[7],p[7],p[6]);
and(gw[6],p[7],g[6]); or(g1[7],gw[6],g[7]);
endmodule
//두번째 단 연결 8bit---------------------------------------------
module kogge2_8bit(p1,g1,p2,g2);
input [7:0] p1,g1;
output [7:2] p2,g2;
wire gw[7:0];
and(p2[2],p1[2],p1[0]);
and(gw[0],p1[2],g1[0]); or(g2[2],gw[0],g1[2]);
and(p2[3],p1[3],p1[1]);
and(gw[1],p1[3],g1[1]); or(g2[3],gw[1],g1[3]);
and(p2[4],p1[4],p1[2]);
and(gw[2],p1[4],g1[2]); or(g2[4],gw[2],g1[4]);
and(p2[5],p1[5],p1[3]);
and(gw[3],p1[5],g1[3]); or(g2[5],gw[3],g1[5]);
and(p2[6],p1[6],p1[4]);
and(gw[4],p1[6],g1[4]); or(g2[6],gw[4],g1[6]);
and(p2[7],p1[7],p1[5]);
and(gw[5],p1[7],g1[5]); or(g2[7],gw[5],g1[7]);
endmodule
//세번째 단 연결 8bit --------------------------------------------
module kogge3_8bit(p2,g2,p3,g3);
input [7:0] p2,g2;
output [7:4] p3,g3;
wire gw[7:0];
and(p3[4],p2[4],p2[0]);
and(gw[0],p2[4],g2[0]); or(g3[4],gw[0],g2[4]);
and(p3[5],p2[5],p2[1]);
and(gw[1],p2[5],g2[1]); or(g3[5],gw[1],g2[5]);
and(p3[6],p2[6],p2[2]);
and(gw[2],p2[6],g2[2]); or(g3[6],gw[2],g2[6]);
and(p3[7],p2[7],p2[3]);
and(gw[3],p2[7],g2[3]); or(g3[7],gw[3],g2[7]);
endmodule
//네번째 단 연결 16bit --------------------------------------------
module kogge4_16bit(p3,g3,p4,g4);
input [15:0] p3,g3;
output [15:8] p4,g4;
wire [7:0] gw;
and(p4[8],p3[8],p3[0]);
and(gw[0],p3[8],g3[0]); or(g4[8],gw[0],g3[8]);
and(p4[9],p3[9],p3[1]);
and(gw[1],p3[9],g3[1]); or(g4[9],gw[1],g3[9]);
and(p4[10],p3[10],p3[2]);
and(gw[2],p3[10],g3[2]); or(g4[10],gw[2],g3[10]);
and(p4[11],p3[11],p3[3]);
and(gw[3],p3[11],g3[3]); or(g4[11],gw[3],g3[11]);
and(p4[12],p3[12],p3[4]);
and(gw[4],p3[12],g3[4]); or(g4[12],gw[4],g3[12]);
and(p4[13],p3[13],p3[5]);
and(gw[5],p3[13],g3[5]); or(g4[13],gw[5],g3[13]);
and(p4[14],p3[14],p3[6]);
and(gw[6],p3[14],g3[6]); or(g4[14],gw[6],g3[14]);
and(p4[15],p3[15],p3[7]);
and(gw[7],p3[15],g3[7]); or(g4[15],gw[7],g3[15]);
endmodule
//다섯번째단 연결 32bit --------------------------------------------
module kogge5_32bit(p4,g4,p5,g5);
input [31:0] p4,g4;
output [31:16] p5,g5;
wire [15:0] gw;
and(p5[16],p4[16],p4[0]);
and(gw[0],p4[16],g4[0]); or(g5[16],gw[0],g4[16]);
and(p5[17],p4[17],p4[1]);
and(gw[1],p4[17],g4[1]); or(g5[17],gw[1],g4[17]);
and(p5[18],p4[18],p4[2]);
and(gw[2],p4[18],g4[2]); or(g5[18],gw[2],g4[18]);
and(p5[19],p4[19],p4[3]);
and(gw[3],p4[19],g4[3]); or(g5[19],gw[3],g4[19]);
and(p5[20],p4[20],p4[4]);
and(gw[4],p4[20],g4[4]); or(g5[20],gw[4],g4[20]);
and(p5[21],p4[21],p4[5]);
and(gw[5],p4[21],g4[5]); or(g5[21],gw[5],g4[21]);
and(p5[22],p4[22],p4[6]);
and(gw[6],p4[22],g4[0]); or(g5[22],gw[6],g4[22]);
and(p5[23],p4[23],p4[7]);
and(gw[7],p4[23],g4[7]); or(g5[23],gw[7],g4[23]);
and(p5[24],p4[24],p4[8]);
and(gw[8],p4[24],g4[8]); or(g5[24],gw[8],g4[24]);
and(p5[25],p4[25],p4[9]);
and(gw[9],p4[25],g4[9]); or(g5[25],gw[9],g4[25]);
and(p5[26],p4[26],p4[10]);
and(gw[10],p4[26],g4[10]); or(g5[26],gw[10],g4[26]);
and(p5[27],p4[27],p4[11]);
and(gw[11],p4[27],g4[11]); or(g5[27],gw[11],g4[27]);
and(p5[28],p4[28],p4[12]);
and(gw[12],p4[28],g4[12]); or(g5[28],gw[12],g4[28]);
and(p5[29],p4[29],p4[13]);
and(gw[13],p4[29],g4[13]); or(g5[29],gw[13],g4[29]);
and(p5[30],p4[30],p4[14]);
and(gw[14],p4[30],g4[14]); or(g5[30],gw[14],g4[30]);
and(p5[31],p4[31],p4[15]);
and(gw[15],p4[31],g4[15]); or(g5[31],gw[15],g4[31]);
endmodule
module pgxor_8bit(p,g,s);
input [7:0] p,g;
output [7:1] s;
xor(s[1],p[1],g[0]); xor(s[2],p[2],g[1]);
xor(s[3],p[3],g[2]); xor(s[4],p[4],g[3]);
xor(s[5],p[5],g[4]); xor(s[6],p[6],g[5]);
xor(s[7],p[7],g[6]);
endmodule
앞으로 할것!
앞에있는 회로들 NAND, NOT,NOR로 바꾸기. 아 언제다해 진짜
'multiplier, adder 연구일지' 카테고리의 다른 글
32bit-Brent Kung-Adder (0) | 2023.03.09 |
---|---|
9주차 (2023.02.20~2023.02.26) 32bit KSA , Final Simulaion (0) | 2023.02.24 |
8주차 (2023. 02.15~2023.02.17) Booth Algorithm, Wallace Tree (0) | 2023.02.22 |
7주차 ( 2023.02.06~2023.02.09 ) (0) | 2023.02.06 |
6주차 (2023. 1.30~2023.02.03) (0) | 2023.01.30 |